Classification of XOR problem with a PNN
Neural Networks course (practical examples) © 2012 Primoz Potocnik
PROBLEM DESCRIPTION: 2 groups of linearly inseparable data (A,B) are defined in a 2-dimensional input space. The task is to define a neural network for solving the XOR classification problem.
Contents
Create input data
close all, clear all, clc, format compact % number of samples of each cluster K = 100; % offset of clusters q = .6; % define 2 groups of input data A = [rand(1,K)-q rand(1,K)+q; rand(1,K)+q rand(1,K)-q]; B = [rand(1,K)+q rand(1,K)-q; rand(1,K)+q rand(1,K)-q]; % plot data plot(A(1,:),A(2,:),'k+',B(1,:),B(2,:),'b*') grid on hold on
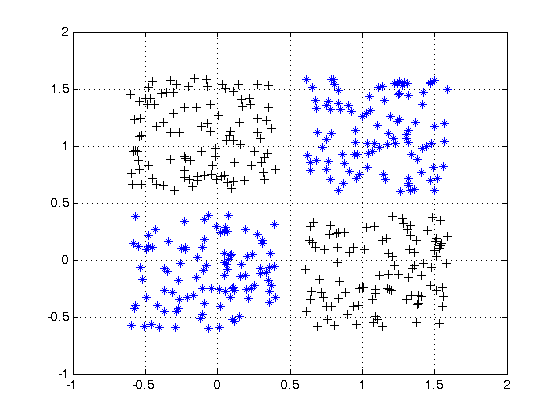
Define output coding
% coding (+1/-1) for 2-class XOR problem
a = 1;
b = 2;
Prepare inputs & outputs for network training
% define inputs (combine samples from all four classes) P = [A B]; % define targets T = [repmat(a,1,length(A)) repmat(b,1,length(B))];
Create a PNN
% choose a spread constant spread = .5; % create a neural network net = newpnn(P,ind2vec(T),spread); % view network view(net)
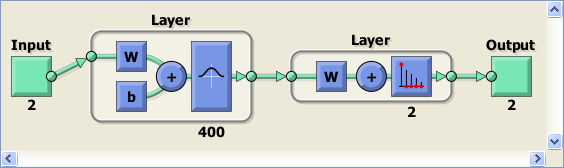
Evaluate network performance
% simulate RBFN on training data Y = net(P); Y = vec2ind(Y); % calculate [%] of correct classifications correct = 100 * length(find(T==Y)) / length(T); fprintf('\nSpread = %.2f\n',spread) fprintf('Num of neurons = %d\n',net.layers{1}.size) fprintf('Correct class = %.2f %%\n',correct) % plot targets and network response figure; plot(T') hold on grid on plot(Y','r--') ylim([0 3]) set(gca,'ytick',[-2 0 2]) legend('Targets','Network response') xlabel('Sample No.')
Spread = 0.50 Num of neurons = 400 Correct class = 100.00 %
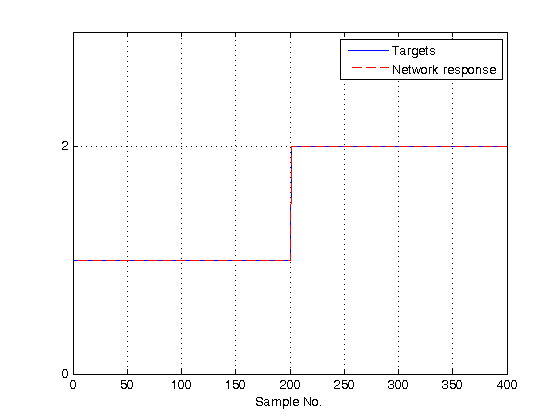
Plot classification result for the complete input space
% generate a grid span = -1:.025:2; [P1,P2] = meshgrid(span,span); pp = [P1(:) P2(:)]'; % simualte neural network on a grid aa = sim(net,pp); aa = vec2ind(aa)-1.5; % convert % plot classification regions based on MAX activation figure(1) ma = mesh(P1,P2,reshape(-aa,length(span),length(span))-5); mb = mesh(P1,P2,reshape( aa,length(span),length(span))-5); set(ma,'facecolor',[1 0.2 .7],'linestyle','none'); set(mb,'facecolor',[1 1.0 .5],'linestyle','none'); view(2)
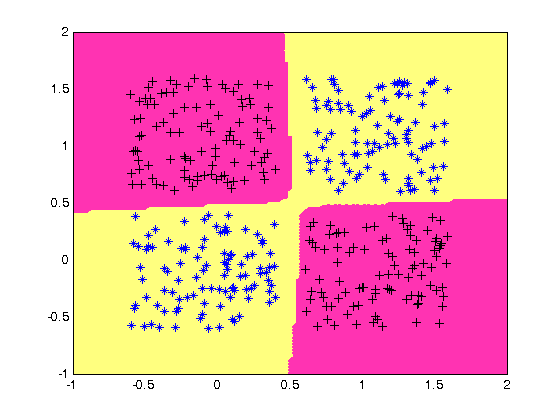
plot PNN centers
plot(net.iw{1}(:,1),net.iw{1}(:,2),'gs')
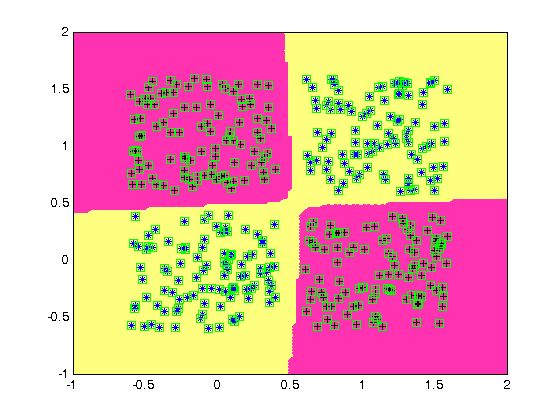