Classification of a 4-class problem with a perceptron
Neural Networks course (practical examples) © 2012 Primoz Potocnik
PROBLEM DESCRIPTION: Perceptron network with 2-inputs and 2-outputs is trained to classify input vectors into 4 categories
Contents
Define data
close all, clear all, clc, format compact % number of samples of each class K = 30; % define classes q = .6; % offset of classes A = [rand(1,K)-q; rand(1,K)+q]; B = [rand(1,K)+q; rand(1,K)+q]; C = [rand(1,K)+q; rand(1,K)-q]; D = [rand(1,K)-q; rand(1,K)-q]; % plot classes plot(A(1,:),A(2,:),'bs') hold on grid on plot(B(1,:),B(2,:),'r+') plot(C(1,:),C(2,:),'go') plot(D(1,:),D(2,:),'m*') % text labels for classes text(.5-q,.5+2*q,'Class A') text(.5+q,.5+2*q,'Class B') text(.5+q,.5-2*q,'Class C') text(.5-q,.5-2*q,'Class D') % define output coding for classes a = [0 1]'; b = [1 1]'; c = [1 0]'; d = [0 0]'; % % Why this coding doesn't work? % a = [0 0]'; % b = [1 1]'; % d = [0 1]'; % c = [1 0]'; % % Why this coding doesn't work? % a = [0 1]'; % b = [1 1]'; % d = [1 0]'; % c = [0 1]';
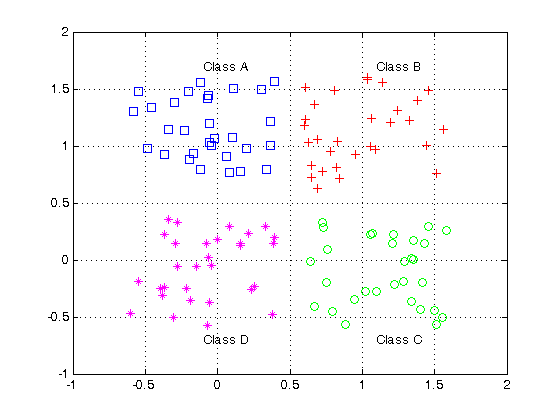
Prepare inputs & outputs for perceptron training
% define inputs (combine samples from all four classes) P = [A B C D]; % define targets T = [repmat(a,1,length(A)) repmat(b,1,length(B)) ... repmat(c,1,length(C)) repmat(d,1,length(D)) ]; %plotpv(P,T);
Create a perceptron
net = perceptron;
Train a perceptron
ADAPT returns a new network object that performs as a better classifier, the network output, and the error. This loop allows the network to adapt for xx passes, plots the classification line, and continues until the error is zero.
E = 1; net.adaptParam.passes = 1; linehandle = plotpc(net.IW{1},net.b{1}); n = 0; while (sse(E) & n<1000) n = n+1; [net,Y,E] = adapt(net,P,T); linehandle = plotpc(net.IW{1},net.b{1},linehandle); drawnow; end % show perceptron structure view(net);
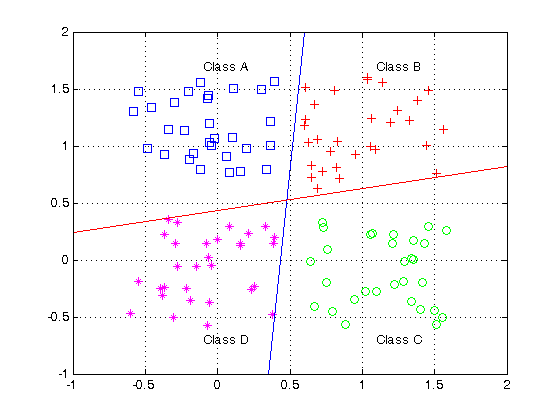
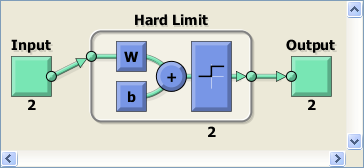
How to use trained perceptron
% For example, classify an input vector of [0.7; 1.2] p = [0.7; 1.2] y = net(p) % compare response with output coding (a,b,c,d)
p = 0.7000 1.2000 y = 1 1